메모
전체 예제 코드를 다운로드 하려면 여기 를 클릭 하십시오.
사용자 지정 상자 스타일 #
이 예제는 사용자 정의 구현을 보여줍니다 BoxStyle
. 사용자 지정 ConnectionStyle
및 ArrowStyle
s는 유사하게 정의할 수 있습니다.
from matplotlib.patches import BoxStyle
from matplotlib.path import Path
import matplotlib.pyplot as plt
사용자 지정 상자 스타일은 직사각형 상자와 "변형" 양을 모두 지정하는 인수를 사용하고 "변형된" 경로를 반환하는 함수로 구현될 수 있습니다. 특정 서명은 다음 중 하나입니다
custom_box_style
.
여기에서 상자 왼쪽에 "화살표" 모양을 추가하는 새 경로를 반환합니다.
그런 다음 사용자 지정 상자 스타일을 에 전달
하여 사용할 수 있습니다 .bbox=dict(boxstyle=custom_box_style, ...)
Axes.text
def custom_box_style(x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box around
it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Mutation reference scale, typically the text font size.
"""
# padding
mypad = 0.3
pad = mutation_size * mypad
# width and height with padding added.
width = width + 2 * pad
height = height + 2 * pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2), (x0, y0),
(x0, y0)],
closed=True)
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle=custom_box_style, alpha=0.2))
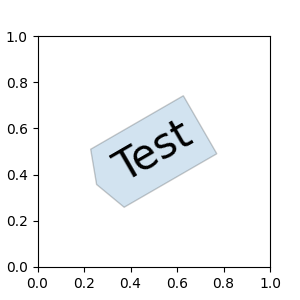
Text(0.5, 0.5, 'Test')
마찬가지로 사용자 지정 상자 스타일을 구현하는 클래스로 구현할 수 있습니다
__call__
.
그런 다음 클래스를 BoxStyle._style_list
dict에 등록하여 상자 스타일을 문자열로 지정할 수 있습니다
. 이 등록은 내부 API에 의존하므로 공식적으로 지원되지 않습니다.bbox=dict(boxstyle="registered_name,param=value,...", ...)
class MyStyle:
"""A simple box."""
def __init__(self, pad=0.3):
"""
The arguments must be floats and have default values.
Parameters
----------
pad : float
amount of padding
"""
self.pad = pad
super().__init__()
def __call__(self, x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box
around it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Reference scale for the mutation, typically the text font size.
"""
# padding
pad = mutation_size * self.pad
# width and height with padding added
width = width + 2.*pad
height = height + 2.*pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2.), (x0, y0),
(x0, y0)],
closed=True)
BoxStyle._style_list["angled"] = MyStyle # Register the custom style.
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle="angled,pad=0.5", alpha=0.2))
del BoxStyle._style_list["angled"] # Unregister it.
plt.show()
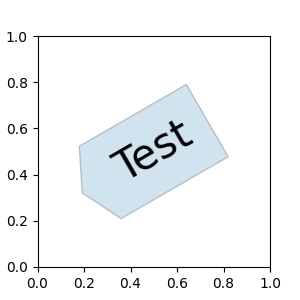